データの保存方法としては、前の記事で紹介したプリファレンスを使う方法などがありますが、
今回は内部記憶領域(ローカル)ファイルを保存する方法を紹介しています。
プリファレンスを使った方式と何が違うかというと、
こちらの方式ではString型やInt型など決まった形ではなく、画像などを大きいデータを保存できます。
今回は簡単なテキストファイルを保存する方法を例に説明してみます。
文字列をテキスト形式にして保存する
ファイル保存には
・FileOutputStreamクラス
・openFileOutput関数
を使用します。
(使用例)
try{ String str = "保存する文字列"; FileOutputStream out = openFileOutput( "test.txt", MODE_PRIVATE ); out.write( str.getBytes() ); }catch( IOException e ){ e.printStackTrace(); }
openFileOutput関数の引数にファイル名とモードを指定します。
第二引数のモードはプリファレンスと同じですね。
MODE_WORLD_READABLE | 他のアプリから読み取り可 |
---|---|
MODE_WORLD_WRITEABLE | 他のアプリから書込み可能 |
MODE_PRIVATE | そのアプリ内でのみ使用可能 |
保存したテキストファイルを読み込む
次は読込みです。今度は
・FileInputStreamクラス
・openFileInput関数
を使います。
改行したデータを一行ずつ読み込む為
BufferedReaderクラスのreadLine関数を利用してデータを取得します。
(使用例)
try{ FileInputStream in = openFileInput( "test.txt" ); BufferedReader reader = new BufferedReader( new InputStreamReader( in , "UTF-8") ); String str = ""; String tmp; while( (tmp = reader.readLine()) != null ){ str = str + tmp + "\n"; } reader.close(); }catch( IOException e ){ e.printStackTrace(); }
保存されたローカルファイルを削除する
最後に削除です。deleteFile( "test.txt" );
たった一行でOKです(^ω^)
入力された文字列をを保存、書き出し・読込み・削除をするサンプルブログラム
import java.io.BufferedReader; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStreamReader; import android.app.Activity; import android.os.Bundle; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.EditText; import android.widget.LinearLayout; public class Sample20120711Activity extends Activity { EditText et; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); LinearLayout ll = new LinearLayout(this); ll.setOrientation(LinearLayout.VERTICAL); setContentView(ll); et = new EditText(this); ll.addView(et); Button btn = new Button(this); btn.setText("ローカルに文字列を保存"); btn.setOnClickListener( new InputClick() ); ll.addView(btn); Button btn2 = new Button(this); btn2.setText("ローカルからテキストを読込み"); btn2.setOnClickListener( new OutputClick() ); ll.addView(btn2); Button btn3 = new Button(this); btn3.setText("ローカルファイルを削除"); btn3.setOnClickListener( new DeleteClick() ); ll.addView(btn3); } class InputClick implements OnClickListener{ @Override public void onClick(View v) { String str = et.getText().toString(); try{ FileOutputStream out = openFileOutput( "test.txt", MODE_PRIVATE ); out.write( str.getBytes() ); }catch( IOException e ){ e.printStackTrace(); } } } class OutputClick implements OnClickListener{ @Override public void onClick(View v) { try{ FileInputStream in = openFileInput( "test.txt" ); BufferedReader reader = new BufferedReader( new InputStreamReader( in , "UTF-8") ); String tmp; et.setText(""); while( (tmp = reader.readLine()) != null ){ et.append(tmp + "\n"); } reader.close(); }catch( IOException e ){ e.printStackTrace(); } } } class DeleteClick implements OnClickListener{ @Override public void onClick(View v) { deleteFile( "test.txt" ); } } }
【実行例】
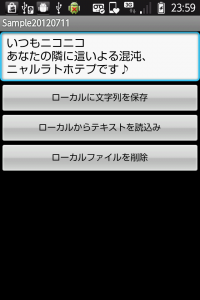